What is a Smart Contract?
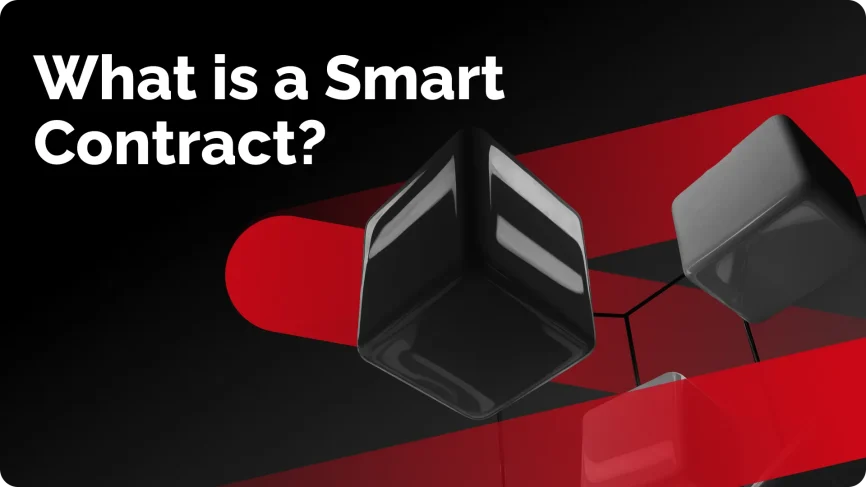
Smart Contracts are self-executing contracts stored on the blockchain where the terms of the agreement between buyer and seller are directly written into lines of code. They automatically enforce and execute the terms and conditions of the contract without the need for intermediaries.
In the context of Smart Contracts, the term ‘Turing Completeness’ is important to know – after all, Smart Contracts require a Turing-complete system to work. It’s essentially a system’s ability to perform any computation that can be described algorithmically, given sufficient time and memory.
A modern computer, for example, is Turing complete because it can run any program you can write. A simple calculator is not Turing complete because it can only perform specific arithmetic operations.
At this point, you’re likely wondering whether blockchains are Turing complete, and if so – do all blockchains have smart contracts?
Bitcoin, much like a calculator, isn’t Turing complete. Indeed, Satoshi Nakomoto aimed to prioritise safe and reliable transaction verification over the flexibility to handle arbitrary computations. The downside was that the deployment of complex decentralised applications (dApps) wasn’t possible, limiting bitcoin’s scope of use.
Ethereum however, proposed by Vitalik Buterin and launched in 2015, was the first blockchain platform designed specifically to support Turing-complete smart contracts.
With unbounded memory (in theory), conditional branching and support for looping constructs and recursion, Ethereum set the foundations for Web3 – an ecosystem not just designed to live on the blockchain, but to thrive.
Ethereum’s Turing completeness meant that developers were able to create complex applications, from decentralised exchanges and lending marketplaces to NFT staking and play to earn (P2E) games.
Today, we’ll be exploring Ethereum’s product of turing completeness – smart contracts, both on a surface and a technical level.
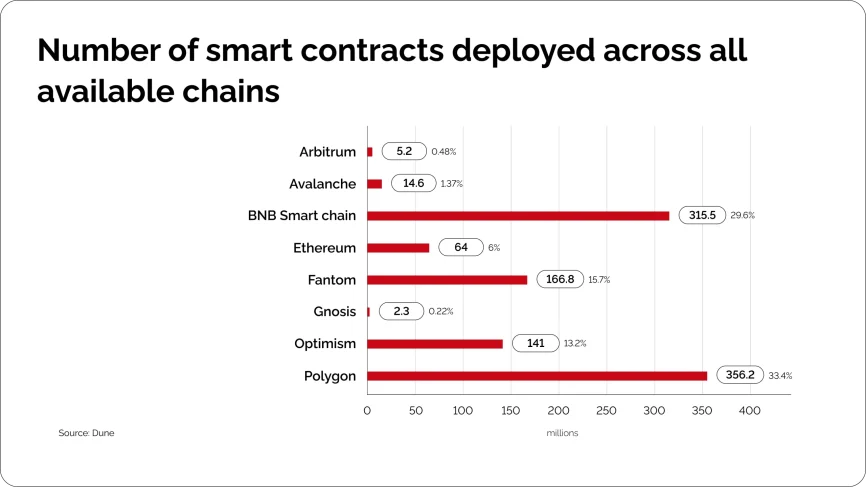
How do Smart Contracts Work?
Imagine a smart contract as a vending machine:
- Before you start, you program the vending machine with certain rules. For example, if you insert $1 and select a specific item, the machine will dispense that item. The rules are clear and visible to everyone.
- Once you follow the rules (insert the money and press the button), the machine automatically performs the action without any need for a middleman or additional approval.
- You don’t need to trust a human cashier to give you the item. As long as the machine is functioning correctly, it will always dispense the item as programmed when the conditions are met.
- Once the machine is set up, it operates exactly as programmed. No one can tamper with the rules or the operation without everyone noticing.
In the same way, smart contracts are computer programs hosted and executed on a blockchain network:
- Developers write the smart contract code, which specifies the rules and actions. The contract code is usually visible on the blockchain, so all parties can see how it works.
- When the predefined conditions are met, the contract automatically executes the specified actions.
- There’s no need to trust a central authority or intermediary; the blockchain ensures the contract is executed as written.
- The blockchain’s decentralised nature makes the contract secure and resistant to tampering.
Just like you trust a machine to dispense your coffee when you insert the right amount of money, you can trust a smart contract to execute its programmed tasks when the specified conditions are met.
Now, it’ll make sense when we define smart contracts as ‘self-executing contracts with the terms of the agreement directly written into code’.
“Smart contracts are ultimately about getting things done automatically and reliably. Once the terms are set in code, there’s no room for misunderstanding or delay.” Artsiom Shapavalau Integration and Support Engineer at CryptoProcessing.com
Advantages of Smart Contracts
The most exciting use case of smart contracts lies in their ability to empower developers to build projects on existing blockchains. Whether it’s a memecoin minting tokens for community trading or a world-renowned restaurant in Downtown New York deploying an NFT loyalty system to advertise their secret menu, smart contracts have significantly accelerated the utility and adoption of blockchain technology.
Importantly, smart contracts have the ability to replace manual processes and paperwork within the legacy system. Aside from boasting improved trust and transparency, immediate execution and immutability, these paper-free alternatives help avoid form-filling errors and ensure the explicit recording of terms and conditions in a way that enables unparalleled savings with storage and backup features.
In terms of what can be built – the possibilities are boundless. For example, in agriculture, weather data fetched by oracles (services that provide smart contracts with external data from the real world) can be used to determine crop insurance payouts. If the data indicates adverse weather conditions, the smart contract automatically releases funds to the insured farmers, eliminating the need for manual intervention.
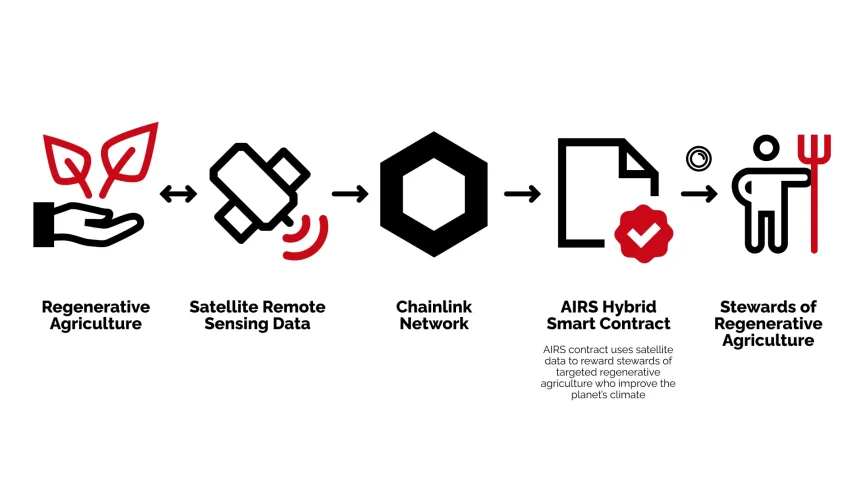
Alternatively, smart contracts could automatically distribute royalties to content creators based on usage data. For instance, musicians can receive payments directly from streaming platforms according to the number of plays their songs receive, ensuring timely and accurate compensation.
Then, there’s Decentralized finance (DeFi) – an industry that wouldn’t exist without smart contracts. Platforms like Aave and Compound utilise smart contracts to facilitate lending and borrowing of cryptocurrencies without the need for intermediaries. These smart contracts manage collateral, calculate interest rates, and ensure that the terms of the loans are adhered to, thereby providing a secure and efficient financial service.
Moreover, decentralised exchanges such as Uniswap and SushiSwap enable users to trade cryptocurrencies directly from their wallets. Smart contracts handle the trading process, matching buy and sell orders, and ensuring the secure transfer of assets between parties without the need for a centralised exchange.
Next, smart contracts can manage rental agreements by automating processes such as rent payments and maintenance requests. For instance, rent can be automatically deducted from a tenant’s account and transferred to the landlord on a specified date each month, while a deposit can be held by the smart contract for the duration of the tenancy, and maintenance requests can be logged and tracked efficiently.
Smart contracts in real estate can also be used to accelerate the handover of property ownership while minimising transaction-related risks.
Governance is also a big talking point – organisations like MakerDAO and Aragon leverage smart contracts to this avail. Token holders can vote on proposals and decisions, with the results being transparently recorded and executed by smart contracts. This decentralised approach to governance ensures that all stakeholders have a say in the organisation’s operations.
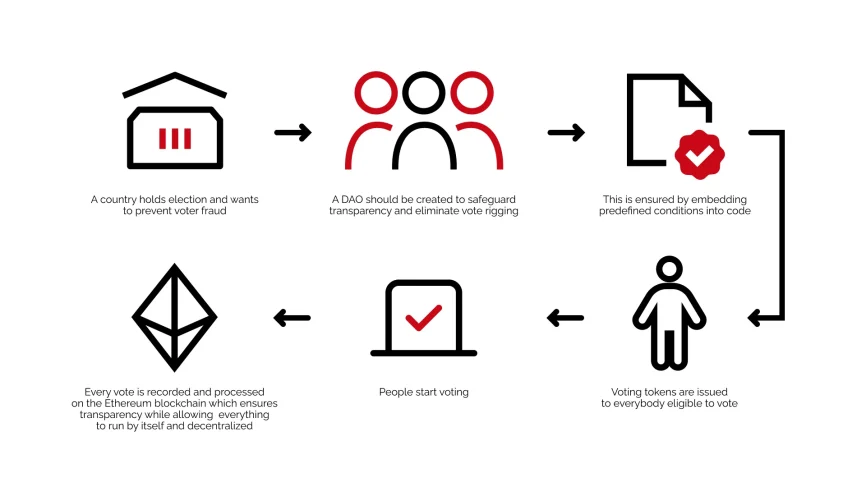
We could elaborate further, but the picture is clear – in adding Turing completeness, smart contracts have transformed blockchain technology, enabling a wide range of applications beyond simple transaction facilitation.
How to write a smart contract?
Let’s imagine a smart contract designed to mint and distribute a new token called NukeCoin when the Doomsday Clock hits midnight.
This token will be sent to nine public addresses belonging to the leaders of each nuclear power in the world. The contract will use an oracle to fetch the time from the Doomsday Clock site. We ought to write this code in Solidity, which is designed to work specifically with the EVM (Ethereum Virtual Machine).
We start with the imports, and I need two. OpenZeppelin, which provides a standard and secure template for creating ERC20 tokens, and the Chainlink Aggregator Interface which is necessary to interact with my oracle (simply, the Doomsday clock).
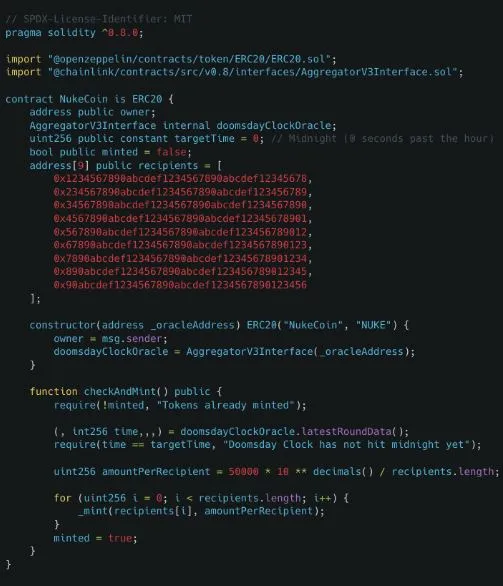
As you can see, the contract inherits from ERC20, which is a standard implementation of the ERC-20 token, which makes it a fungible (stackable) asset. We then have our state variables listed, and below, I’ve inserted an array of 9 recipient addresses.
Regarding the constructor, I’m initialising the contract with the oracle address and setting the token name and symbol. I’ve set the owner of the contract to me, hence ‘msg.sender’.
The contract integrates with a Chainlink oracle (AggregatorV3Interface) to get the Doomsday Clock time. Using Chainlink is key to access external data, in this case, it fetches the current time from the Doomsday Clock site periodically.
Then, I have to designate the Check and Mint Function. This function checks the oracle for the Doomsday Clock time. If it is midnight, it mints 50,000 tokens divided equally among the nine recipients.
Lastly, the target time is set to midnight (0 seconds past the hour), which is when the contract knows to activate. The boolean ‘minted’ is put in place to ensure tokens are minted only once.
It’s now time to test. To deploy the NukeCoin smart contract on a testnet, we will install MetaMask and obtain testnet Ether. Then, we will open Remix IDE and create a new file into which we will paste the contract code.
We can then deploy our contract to the mainnet once we have obtained sufficient ether for gas fees. If we were to deploy such a contract now, I’d guess that the fees would be no more than 150 dollars.
Provided this is all set up correctly when the Doomsday Clock hits midnight and the nukes are launched, the oracle sends this information to the smart contract. The smart contract automatically mints 50,000 NukeCoins. The newly minted tokens are then distributed equally to the nine specified public addresses of the nuclear powers’ leaders.
If nothing else, provided they have notifications set up, it’s a good way of letting our leaders know to head to the space-port.
Final Thoughts
The flexibility and power of Turing complete smart contracts have paved the way for a new era of blockchain utility, transforming how we interact with digital assets and decentralised services. By leveraging the capabilities of the EVM, developers can implement any algorithmic logic, allowing for the creation of applications that were previously impossible on blockchain technology.
As we explored, the process of writing and deploying smart contracts involves defining clear rules and conditions, integrating with oracles for real-world data, and ensuring security and efficiency in execution.
The practice could offer a slew of benefits. For example, in the legal industry, the use of smart contracts offers increased efficiency and reduced costs. These benefits may need to be fed through customs, however, due to complexities related to legal enforceability and regulatory compliance.
My example of the NukeCoin contract, while humorous and very basic, demonstrates how smart contracts can be used for specific scenarios, combining blockchain’s decentralisation with external data sources (oracles, as discussed) to automate complex tasks.
The potential for smart contracts is vast, with applications in finance, real estate, supply chain management, gaming, and beyond. As blockchain technology continues to evolve, the role of smart contracts will undoubtedly expand, driving further innovation and adoption across various industries.
At CryptoProcessing, we cover a range of topics to equip you with the knowledge needed for our gradual transition to Web3. Browse our topics here.
Alternatively, if you represent a company interested in learning how we empower businesses to accept cryptocurrencies through our outstanding crypto processing gateway, fill out a request form on our site, and a manager will get back to you promptly.
Frequently Asked Questions (FAQ)
1. How do I create a smart contract?
To create a smart contract, you need to follow these steps:
- Choose a Blockchain Platform: Select a platform that supports smart contracts, such as Ethereum.
- Learn a Smart Contract Language: Familiarise yourself with a programming language like Solidity (for Ethereum).
- Write the Contract: Write the code for your smart contract, defining the rules and conditions.
- Test the Contract: Use test networks and tools to ensure your contract works as intended.
- Deploy the Contract: Deploy your smart contract on the blockchain network.
- Interact with the Contract: Use interfaces like web apps or command-line tools to interact with your contract.
2. How are smart contracts executed?
Smart contracts are executed automatically by the blockchain network. Here’s how it works:
- Trigger: An event or transaction triggers the smart contract.
- Verification: The network nodes verify that the conditions specified in the contract are met.
- Execution: If conditions are met, the contract executes the predefined actions.
- Recording: The transaction and its outcome are recorded on the blockchain, ensuring transparency and immutability.
3. What are the major parts of a smart contract?
The major parts of a smart contract include:
- Conditions and Rules: Define what the contract does and the conditions under which it operates.
- Functions: Contain the actions that the contract can perform.
- Data Storage: Variables that hold data relevant to the contract, stored on the blockchain.
- Events: Emit signals to external listeners when certain conditions are met or actions are performed.
4. What are the major benefits of smart contracts?
Smart contracts offer several benefits:
- Automation: Execute transactions automatically when conditions are met, reducing the need for intermediaries.
- Transparency: All parties can see the contract terms and verify actions taken.
- Security: Blockchain’s cryptographic methods ensure that the contract is tamper-proof.
- Efficiency: Streamline processes and reduce the time required for contract execution.
- Cost Reduction: Eliminate intermediaries, reducing costs associated with transaction fees and legal processes.
5. What is the most popular smart contract blockchain?
The most popular smart contract blockchain is Ethereum. Ethereum pioneered smart contract functionality and continues to be the leading platform for decentralised applications (dApps) and smart contracts, thanks to its robust developer community and extensive ecosystem.